Canvas Draw 2D
What is it?
Canvas Draw 2D is an online vector graphics editor.
The editor was created specifically for creating vector art for the HTML 2D Canvas API in the form of native Javascript code. The editor exports Javascript modules by default.
Why?
I've been working on the editor off and on over the last couple of years, and I've used it to generate art for some of the games over here on itch.io. While I could use existing vector editors such as Inkscape/Illustrator or Blender to create art for HTML Canvas, I wanted a way to get the art into my games without having to rely on some kind of third-party importer. I also wanted some additional features such as nested transformations with grid snapping, as well as the ability to use all the features in HTML Canvas such as curves and dashed lines. Nested transforms allows for things such as rotating joints on characters, and nested snapping allows for keeping integer positions even inside rotated and scaled children (a feature I wish Blender supported too).
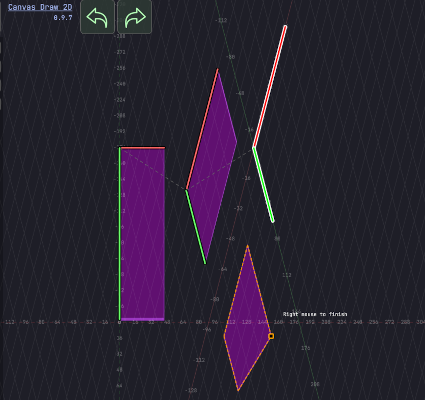
The exported code is minimalistic and dependency-free, making it easy to just copy-paste into your own code.
Editor:
The editor supports most of the major features of the Canvas API:
- The scene objects are drawn from the top downwards. Objects higher up in the scene explorer are drawn on top of lower objects.
- Line dash can be added by inputting the comma-separated numbers in "Line dash".
- Curved lines are created by shift+dragging "handles" from existing lines, and removed again by ctrl+click to delete the handles.
- The text font format is in the same format as in the Canvas API.
- Nested children inherit the transform of the parents.
- Object styles (color, line width etc.) can be copied to other objects using the copy style button.
- The scene or objects can be exported and imported as JSON files.
- Importing a scene replaces the current scene.
- Importing an object inserts the object as a child of the selected object.
- The transform object transforms its children but doesn't draw anything. This type of object can be used as pivot points/joints.
- Like in Blender, objects can be scaled, moved and rotated using the keys S, G and R.
- Objects can be duplicated using shift+D.
- Focus the selected object using Z.
- Objects can be re-parented by dragging and dropping it in the scene explorer. This doesn't update the object's transform.
- Grid snapping can be enabled and disabled using caps-lock or by holding shift.
- The grid size can be adjusted.
- For the best performance, avoid having too many deep branches in the scene tree, as each new branch forces a complete re-transform from the scene root back to branch level.
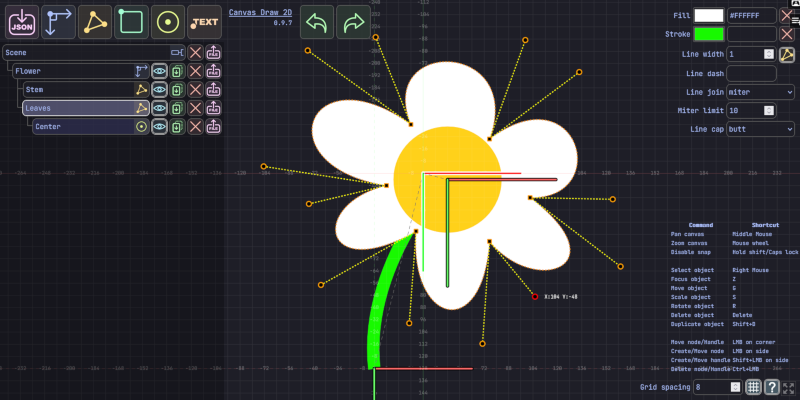
Export format:
The editor supports two types of export formats:
The JSON format is used to store the original objects. The JSON files can be imported back into the editor at a later stage. Non-scene objects can also be imported into existing scenes. Imported scenes replace the current scene.
The Javascript Module format contains the rendering code for drawing the image in a HTML Canvas. The following is an example module exported by the editor:
// Canvas 2D renderer for object "Flower" const d2r = Math.PI / 180, noDash = []; function resetTransform(ctx) { ctx.setTransform(1, 0, 0, 1, 0, 0); } export default function draw(ctx, setRootTransform = resetTransform) { // Transform root setRootTransform(ctx); // Transform "Leaves" ctx.translate(32, -128); // Draw "Leaves" ctx.fillStyle = "#FFFFFF"; ctx.beginPath(); ctx.moveTo(-24, 8); ctx.bezierCurveTo(-104.51, -4.54, -75.65, -80.51, -8, -32); ctx.bezierCurveTo(-31.1, -89.22, 67.01, -87.36, 43.52, -22.46); ctx.bezierCurveTo(102.34, -66.36, 124.28, 17.03, 70.14, 15.94); ctx.bezierCurveTo(129.4, 61.06, 73.34, 80.83, 43.52, 44.61); ctx.bezierCurveTo(38.91, 111.68, -9.09, 98.24, -4.61, 37.95); ctx.bezierCurveTo(-66.95, 73.15, -74.82, 20.03, -24, 8); ctx.closePath(); ctx.fill(); }
Some things to keep in mind:
- The "setRootTransform" function parameter is used to reset the 2D context transformation matrix. This function may be called multiple times depending on how many branches the image has (as this forces the drawing to start from the top again). You would override this function if you wanted to move and rotate an object from a non-zero position, such as moving a player sprite.
- The code in the draw function can be imported and used in your own code (see the downloadable sample), or you can copy-paste the code from the draw function directly without using the module. There are no external dependencies, and the code is very basic and easily understandable.
- The canvas settings (line width, fill color etc.) are reset the first time they are used, as their previous settings can not be assumed to be the defaults. For example, the user may have used another fill color before calling the draw function. You can manually remove this code if you want to optimize the drawing.
- The transformations are labeled with the name of the transformed object. This can be useful if you want to animate a transformation by adding your own variables to it.
License and disclaimer:
The generated code is your own, and is provided without warranty of any kind. Please understand the risks associated with using code generators in Javascript, and do not import JSON files from unverified sources.
Any exported template code (the parts which are hard-coded) is licensed under CC0.
Assets:
The font used in the editor is JetBrains Mono under the Open Font License.
Bug reports:
Please report any bugs or issues with the exported code or feature requests in the comments or in a private message.
Status | In development |
Category | Tool |
Platforms | HTML5 |
Rating | Rated 5.0 out of 5 stars (2 total ratings) |
Author | Donitz |
Tags | 2D, canvas, Generator, graphics, html, html5, sourcecode, Vector, vector-editor |
Leave a comment
Log in with itch.io to leave a comment.